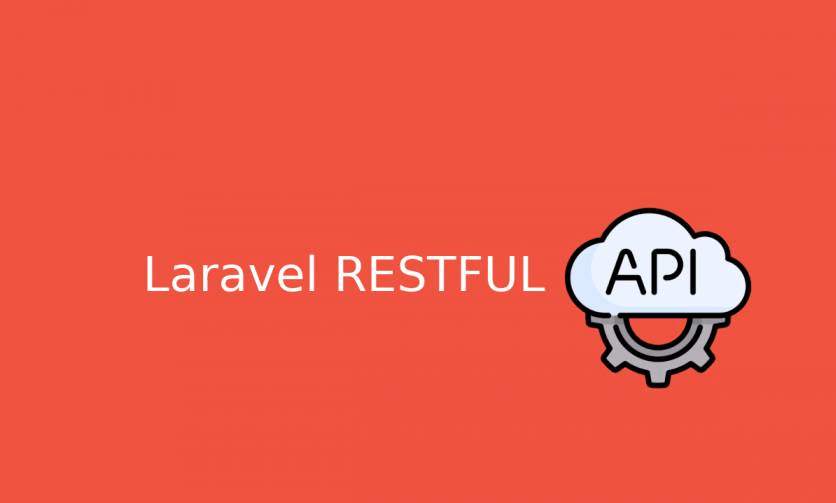
In this tutorial, we will explore how to implement a robust and secure RESTful API using Laravel, a powerful PHP framework. We will leverage Laravel’s built-in features, such as routing, controllers, and middleware, to create an API that follows RESTful principles. Throughout the tutorial, we will cover essential topics like authentication, rate limiting, pagination, and versioning, ensuring that your API meets the highest standards of security and performance.
Prerequisites: To follow along with this tutorial, you should have a basic understanding of PHP and Laravel. Familiarity with RESTful API concepts and HTTP protocols will also be beneficial. Make sure you have Laravel and its dependencies installed on your system.
Setting up the Project: To begin, let’s set up a new Laravel project. Open your terminal and run the following command:
composer create-project --prefer-dist laravel/laravel rest-api-tutorial
This command will create a new Laravel project named “rest-api-tutorial.”
Creating API Routes: Laravel provides a concise and expressive way to define routes. Open the routes/api.php
file and define your API routes. For example:
use App\Http\Controllers\API\UserController;
Route::middleware('auth:api')->group(function () {
Route::get('users', [UserController::class, 'index']);
Route::get('users/{id}', [UserController::class, 'show']);
Route::post('users', [UserController::class, 'store']);
Route::put('users/{id}', [UserController::class, 'update']);
Route::delete('users/{id}', [UserController::class, 'destroy']);
});
In this example, we have defined routes for retrieving users, creating a new user, updating user details, and deleting a user. The auth:api
middleware ensures that these routes are protected and require authentication.
Creating the UserController: Next, let’s create the UserController
to handle these API requests. Run the following command in your terminal:
php artisan make:controller API/UserController --api
This command will generate a new controller named UserController
in the API
namespace with the necessary boilerplate code for an API controller.
Implementing Authentication: To secure your API, Laravel provides various authentication mechanisms. In this tutorial, we will use Laravel’s built-in token-based authentication system. Let’s generate the migration for the api_tokens
table by running the following command:
php artisan migrate
To authenticate users and generate tokens, add the following code to the User
model:
use Laravel\Sanctum\HasApiTokens;
class User extends Authenticatable
{
use HasApiTokens;
// ...
}
With this setup, Laravel will automatically generate a token for each authenticated user.
Rate Limiting: To prevent abuse and protect your API’s resources, implementing rate limiting is crucial. Laravel provides a simple way to configure rate limiting. Open the app/Http/Kernel.php
file and add the throttle:60,1
middleware to the $middlewareGroups
array in the api
group. This sets a limit of 60 requests per minute with a 1-second delay between requests.
Pagination: When dealing with large datasets, paginating API responses enhances performance and improves the user experience. Laravel makes pagination effortless. In your UserController
, modify the index
method as follows:
public function index()
{
$users = User::paginate(10);
return response()->json($users);
}
Now, when accessing the /users
endpoint, the API will return paginated results with ten users per page.
Versioning: API versioning allows you to introduce breaking changes without affecting existing clients. Let’s implement API versioning using Laravel’s routing capabilities. Create a new folder named v1
inside the app/Http/Controllers/API
directory. Move the UserController.php
file into the v1
folder. Then, modify the UserController
namespace and class declaration accordingly:
namespace App\Http\Controllers\API\v1;\
class UserController extends Controller
{
// ...
}
Next, define a new route group in the routes/api.php
file for versioning:
Route::prefix('v1')->group(function () {
Route::middleware('auth:api')->group(function () {
Route::apiResource('users', 'App\Http\Controllers\API\v1\UserController');
});
});
This example sets up versioning for the user-related routes under the /v1/users
endpoint.
Woohoo! You’ve successfully implemented a robust and secure RESTful API using Laravel. We covered essential topics like authentication, rate limiting, pagination, and versioning. You can now extend this foundation to build powerful APIs tailored to your specific requirements. Laravel’s flexibility and extensive documentation make it a fantastic choice for developing APIs. Happy coding!
Lyron Foster is a Hawai’i based African American Author, Musician, Actor, Blogger, Philanthropist and Multinational Serial Tech Entrepreneur.