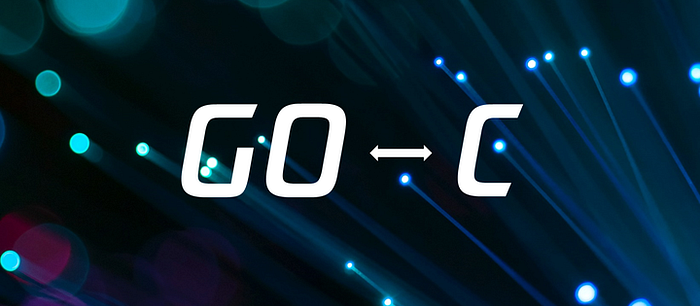
The Go programming language, commonly known as Golang, is designed to be simple and efficient. However, there are times when you might need to leverage existing C libraries or embed Go into other languages. This tutorial dives deep into the world of CGO — Go’s gateway to the world of C and vice versa.
1. What is CGO?
CGO enables the creation of Go packages that call C code. By using CGO with Go, you get the power to use existing C libraries and also potentially optimize performance-critical portions of your application.
To use CGO, you need to have C development tools installed on your machine. This typically includes a C compiler like gcc
.
2. Calling C Code from Go
2.1 Basic Interoperability
Here’s a simple example of how to call C code from Go:
/*
#include <stdio.h>
*/
import "C"
func main() {
C.puts(C.CString("Hello from C!"))
}
In the code above:
- The
import "C"
is a special import that represents the C space. - The C code is wrapped in a Go multi-line string comment.
C.puts
calls the C functionputs
.
2.2 Using C Structs and Functions
Suppose you have the following C code:
// mathfuncs.c
#include "mathfuncs.h"
int add(int a, int b) {
return a + b;
}
// mathfuncs.h
int add(int a, int b);
You can call the add
function from Go like this:
/*
#cgo CFLAGS: -I .
#cgo LDFLAGS: -L . -lmathfuncs
#include "mathfuncs.h"
*/
import "C"
import "fmt"
func main() {
a, b := 3, 4
result := C.add(C.int(a), C.int(b))
fmt.Printf("%d + %d = %d\n", a, b, int(result))
}
3. Embedding Go into Other Languages
3.1 Exporting Go Functions for C
To make Go functions accessible from C (and by extension, other languages), you can use the //export
directive.
// export.go
package main
import "C"
import "fmt"
//export SayHello
func SayHello(name *C.char) {
fmt.Printf("Hello, %s!\n", C.GoString(name))
}
func main() {}
After compiling this Go code into a shared library, the exported SayHello
function can be called from C.
3.2 Calling Go from C
After creating a shared library using go build -o mylib.so -buildmode=c-shared export.go
, you can use it in C:
// main.c
#include "export.h"
int main() {
SayHello("CGO");
return 0;
}
Compile with gcc main.c -L . -lmylib -o output
.
4. Best Practices
- Safety First: Remember that CGO can bypass Go’s memory safety. Always ensure your C code is safe and doesn’t have leaks or buffer overflows.
- Performance: Crossing the Go-C boundary can be expensive in terms of performance. Avoid frequent transitions if possible.
- Error Handling: Ensure you handle errors gracefully, especially when transitioning between languages.
CGO offers a powerful way to bridge Go with C, allowing you to leverage existing libraries and functionalities. With careful planning and understanding of both Go and C ecosystems, you can use CGO effectively and safely.
Lyron Foster is a Hawai’i based African American Author, Musician, Actor, Blogger, Philanthropist and Multinational Serial Tech Entrepreneur.